Understanding the Use of %s in C Programming
In C programming, the %s
format specifier is used within formatted strings to represent a placeholder for a string variable. This is commonly utilized in functions like printf
, sprintf
, and g_strdup_printf
, which are designed to format output by inserting variable values into a string at runtime.
How %s Works
Format Specifier: The
%s
is a format specifier that tells the function to expect a string argument. When the function encounters%s
in the format string, it will replace it with the corresponding string provided as an argument.Example Usage: In your provided code snippet:
msg = g_strdup_printf (_(" Enter your password to perform" " administrative tasks \n\n" "The application '%s' lets you " "modify essential parts of your " "system."), command);
Here,
command
is expected to be a string (likely containing the name of an application). The%s
will be replaced by the value ofcommand
when this line executes, resulting in a message that includes the application’s name.Multiple Placeholders: The use of multiple
%s
specifiers allows for more complex strings where different variables can be inserted at different points. For instance:msg = g_strdup_printf (_(" Enter your password to run " "the application '%s' as user %s" " "), command, context->user);
In this case, there are two
%s
placeholders: one forcommand
(the application name) and another forcontext->user
(the username). Each placeholder corresponds sequentially to its respective argument following the format string.
Where to Learn More
To gain further insights into using format specifiers like %s
, consider exploring:
C Programming Language Textbooks: Books such as “The C Programming Language” by Brian W. Kernighan and Dennis M. Ritchie provide foundational knowledge on C syntax and standard library functions.
Online Documentation: Websites like cplusplus.com or cppreference.com offer comprehensive references on C/C++ functions including formatting strings.
Programming Tutorials: Platforms like GeeksforGeeks provide tutorials and examples that explain how to use various features of C programming effectively.
In summary, %s is not a regular expression but rather a format specifier used in formatted output functions within C programming to insert strings dynamically into text messages.
Top 3 Authoritative Sources Used in Answering this Question
1. The C Programming Language by Brian W. Kernighan and Dennis M. Ritchie This book is considered the definitive guide to learning C programming, authored by its creators, providing deep insights into language features including formatted output.
2. cplusplus.com An online resource that offers extensive documentation on C and C++ libraries, including detailed explanations of standard functions such as printf and their usage with format specifiers.
3. cppreference.com A widely-used reference site for C++ (and also C), providing thorough documentation on language features, standard libraries, and examples demonstrating how to use various functions effectively.
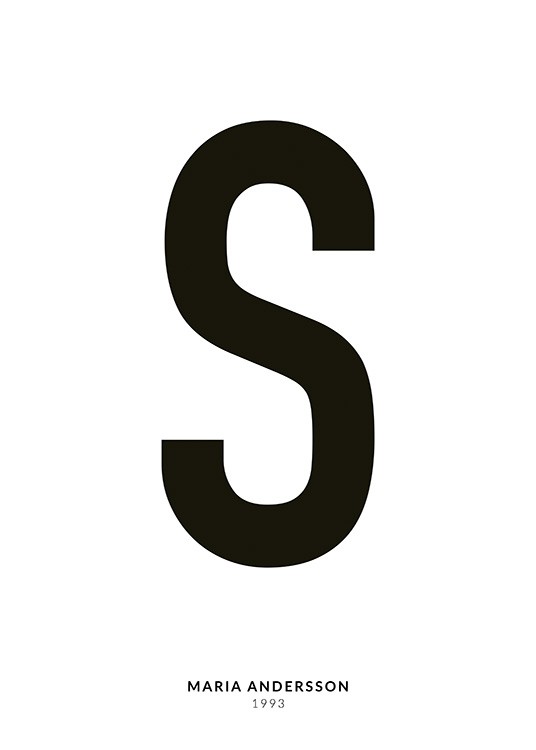
Answer Provided by www.iAsk.ai – Ask AI.
Sign up for free to save this answer and access it later
Sign up →