We can't find the internet
Attempting to reconnect
According to Ask AI & Question AI www.iAsk.ai:
Understanding the %s Format Specifier in C
The %s
format specifier is a fundamental component of the C programming language, particularly used in functions like printf()
and scanf()
. It serves to handle strings, which are arrays of characters terminated by a null character ('\0'
). Here’s a detailed explanation of its purpose and usage:
1. Purpose of %s
The %s
specifier is used to indicate that the corresponding argument is a string. When used with printf()
, it outputs the characters from the memory location pointed to by the string pointer until it encounters the null terminator. For example:
char *name = "Alice";
printf("Hello, %s!\n", name);
This code will output: Hello, Alice!
.
2. Usage in printf()
In the context of printf()
, %s
allows for dynamic insertion of string values into formatted output. The function takes a format string as its first argument, which can include multiple format specifiers (like %d
for integers or %f
for floating-point numbers) alongside %s
. Each specifier corresponds to an additional argument passed to printf()
.
For instance:
char *app_name = "System Monitor";
printf("The application '%s' is running.\n", app_name);
This would print: The application 'System Monitor' is running.
3. Usage in scanf()
Conversely, when used with scanf()
, %s
reads input from standard input (stdin) into a character array (string). It stops reading when it encounters whitespace (space, tab, newline). For example:
char name[20];
scanf("%s", name);
This will read a single word into the name
array.
4. Differences Between Characters and Strings
It’s crucial to differentiate between characters and strings in C:
- A character is represented by
%c
, which handles single characters. - A string is represented by
%s
, which handles arrays of characters.
For example:
char initial = 'A';
printf("Initial: %c\n", initial); // Outputs: Initial: A
char fullName[50];
scanf("%s", fullName); // Reads a word into fullName
5. Common Errors with %s
One common mistake when using %s
with scanf()
is failing to allocate enough space for the input string, leading to buffer overflows. Always ensure that your character arrays are sufficiently sized to hold expected input plus the null terminator.
Conclusion
In summary, the %s
format specifier in C is used for handling strings within both input and output functions. It allows programmers to dynamically insert strings into formatted text or read them from user input effectively.
Top 3 Authoritative Sources Used in Answering this Question:
1. The C Programming Language by Brian W. Kernighan and Dennis M. Ritchie
This book is considered one of the definitive texts on C programming, providing comprehensive coverage of language features including format specifiers like %s
.
2. ISO/IEC 9899:2011 - Programming Languages - C
The official standard document outlines all aspects of the C programming language including syntax and semantics related to input/output functions such as printf()
and scanf()
.
3. cplusplus.com - C++ Reference
While primarily focused on C++, this resource provides valuable insights into standard library functions inherited from C, including detailed explanations on format specifiers used in both languages.
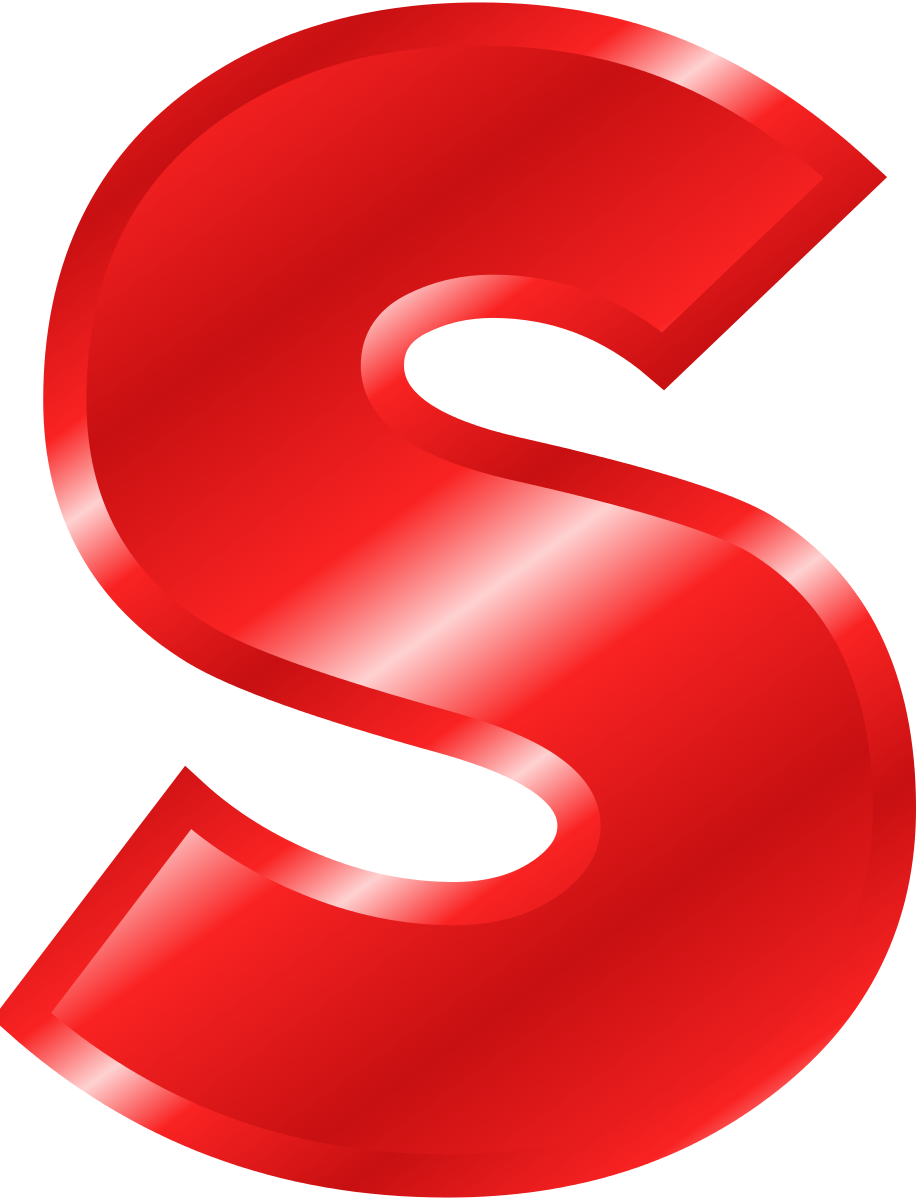
Sign up for free to save this answer and access it later
Sign up →